Intercom is a real-time business messaging platform that lets you bring together and manage all your customer life cycle activities in a single platform.
Getting started
RudderStack supports sending event data to Intercom via the following connection modes:
Connection mode | Web | Mobile | Server |
---|---|---|---|
Device mode | Supported | Supported | - |
Cloud mode | Supported | Supported | Supported |
https://widget.intercom.io/
domain. Based on your website's content security policy, you might need to allowlist this domain to load the Intercom SDK successfully.Once you have confirmed that your source platform supports sending events to Intercom, follow these steps:
- From your RudderStack dashboard, add the source. Then, from the list of destinations, select Intercom.
- Assign a name to the destination and click Next.
Connection settings
To successfully configure Intercom as a destination, you will need to configure the following settings:
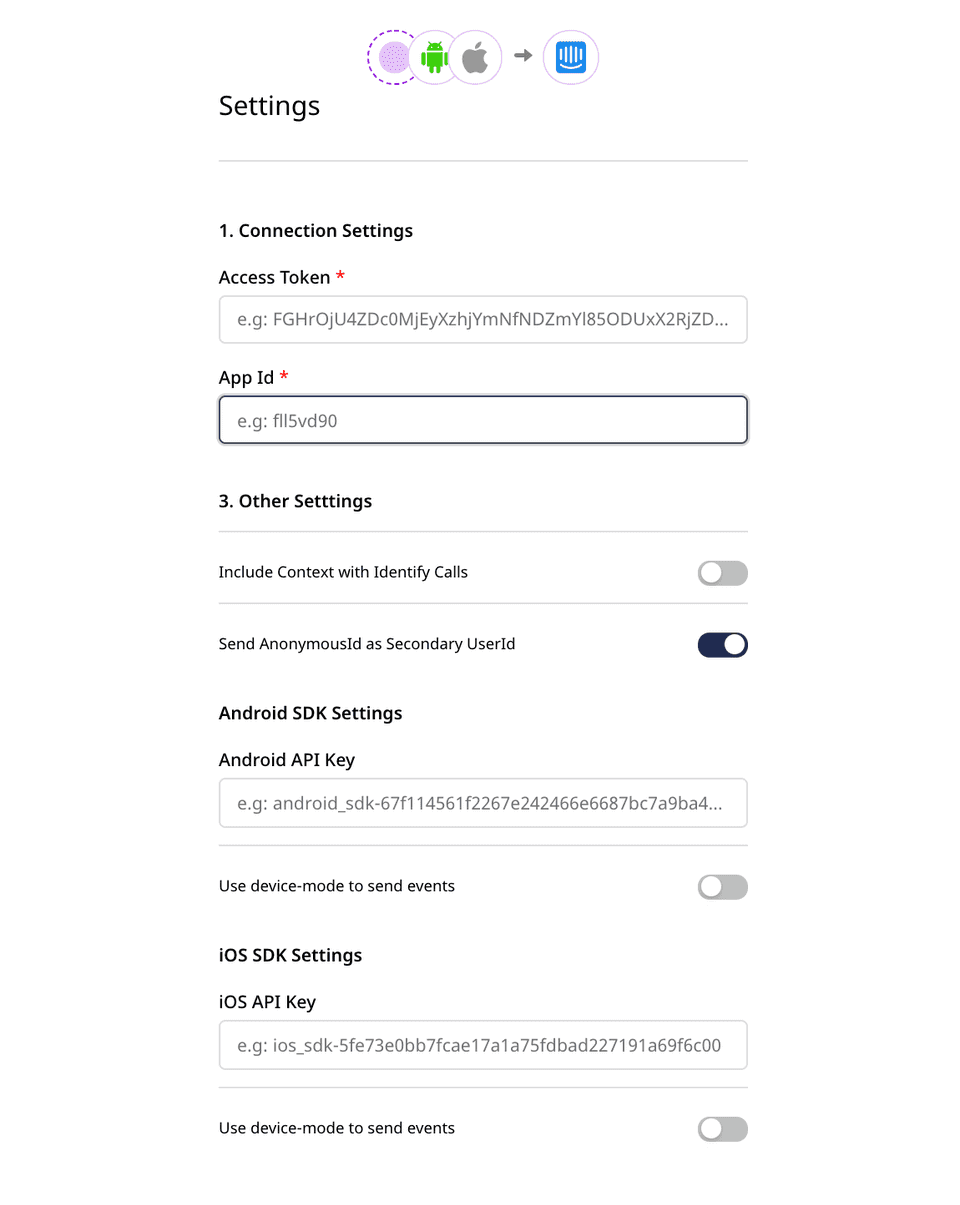
- Access Token: Enter your Intercom API access token. You can obtain the token by going to your Intercom dashboard and navigating to Settings > Apps & Integrations > Developer Hub. Then, select your app and go to Configure > Authentication.
- App ID: The Intercom app ID is required for sending events via the web and mobile SDKs. You can get this ID from your Intercom dashboard by going to Settings > Installation and selecting the relevant platform.
- Android API Key / iOS API Key: This is required for sending events from your mobile apps to Intercom. You can get it from your Intercom dashboard by going to Settings > Installation and selecting the relevant platform.
- Include Context with Identify Calls: Enable this option to send the user context details to Intercom in the
identify
calls. - Send AnonymousId as Secondary UserId: Enable this option to send
anonymousId
as the user ID to Intercom when theuserId
is absent from the event payload.
Adding device mode integration
Depending on your platform of integration, follow the steps below to add Intercom to your project:
Your Android app must be on version 5.0 (API level 21) or higher for RudderStack to be able to send events to Intercom.
Once confirmed, follow these steps to add Intercom to your Android project:
- In your app-level
build.gradle
file, add the followingrepository
:repositories {mavenCentral()} - Then, add the following under
dependencies
:// Rudder core sdk and intercom extensionimplementation 'com.rudderstack.android.sdk:core:1.0.2'implementation 'com.rudderstack.android.integration:intercom:0.1.1'// intercom core sdkimplementation 'io.intercom.android:intercom-sdk-base:6.+'// gsonimplementation 'com.google.code.gson:gson:2.8.6'// FCMimplementation 'com.google.firebase:firebase-messaging:20.2.0' - Change the initialization of your RudderStack client with the following:val rudderClient:RudderClient = RudderClient.getInstance(this,WRITE_KEY,RudderConfig.Builder().withDataPlaneUrl(DATA_PLANE_URL).withLogLevel(RudderLogger.RudderLogLevel.DEBUG) // optional.withFactory(IntercomIntegrationFactory.FACTORY).build())
To add the RudderStack iOS SDK to your project, follow these steps:
- Add the required pod followed by
pod install
:pod 'Rudder-Intercom' - Initialize the client as follows:RSConfigBuilder *builder = [[RSConfigBuilder alloc] init];[builder withDataPlaneUrl:DATA_PLANE_URL];[builder withFactory:[RudderIntercomFactory instance]];[builder withLoglevel:RSLogLevelDebug]; // optional[RSClient getInstance:WRITE_KEY config:[builder build]];
- Add a Privacy - Photo Library Usage Description entry to your
Info.plist
. This is required by Apple for applications that can access the photo library.Users will be prompted for the permission to access the photo library only when they tap the button to upload their images.
Identify
The identify
call captures the details about a visiting user.
A sampleidentify
call looks like the following code snippet:
rudderanalytics.identify("1hKOmRA4GRlm", { name: "Alex Keener", email: "alex@example.com", company: { id: "group01", name: "Tech Group", }, createdAt: "Mon May 19 2019 18:34:24 GMT+0000 (UTC)",})
Using identify
calls
You can use the identify
call to create or update user information in Intercom, as explained below:
- Create/update a user: When you make an
identify
call, RudderStack creates or updates the user in Intercom using their Users API. - Remove users from a company: To remove users from a company, you can pass
remove: true
inside thecompany
object, as shown:
rudderanalytics.identify("1hKOmRA4GRlm", { name: "Alex Keener", email: "alex@example.com", company: { id: "group01", name: "Tech Group", remove: true }, createdAt: "Mon May 19 2019 18:34:24 GMT+0000 (UTC)",})
- Unsubscribe users: To unsubscribe users from emails, you can pass
unsubscribedFromEmails: true
inside thecontext
object, as shown:
rudderanalytics.identify("1hKOmRA4GRlm", { name: "Alex Keener", email: "alex@example.com", company: { id: "group01", name: "Tech Group", }, unsubscribedFromEmails: true, createdAt: "Mon May 19 2019 18:34:24 GMT+0000 (UTC)",})
RudderStack does not support Intercom's Last Seen feature currently.
Traits mapping
The following table lists the mapping of the RudderStack traits to the Intercom properties:
RudderStack trait | Intercom iOS property | Intercom Android property |
---|---|---|
traits.userId | user_id | user_id |
traits.email | email | email |
traits.name | name | name |
traits.phone | phone | phone |
traits.company | company | company |
traits.createdAt | signedUpAt | signed_up_at |
Collecting identify
context
If you enable the Include Context with Identify Calls option in the RudderStack dashboard, RudderStack collects the following contextual properties through its mobile libraries (if available):
device.type
device.model
device.manufacturer
os.name
os.version
app.name
app.version
Identity verification
Intercom's identity verification feature ensures the privacy of the conversations between you and your users. It also makes sure that one user cannot impersonate another.
RudderStack supports the identity verification feature for the events sent through the web device mode.
To enable the identity verification feature for web device mode, you can pass user_hash
within the integrations object, as shown in the following snippet:
rudderanalytics.identify( "1hKOmRA4GRlm", { name: "Alex Keener", country: "USA" }, { Intercom: { user_hash: "9c56cc51b374c3ba189210d5b6d4bf57790d351c96c47c02190ecf1e430635ab", }, });
The user_hash
is a SHA256 hash of your Intercom API secret and the userId
. Note that this hash is not based on the user's email.
To obtain your Intercom API secret, go to your Intercom dashboard and navigate to Settings > Apps & Integrations > Developer Hub. Then select your app and go to Configure > Basic information. You will find the API secret listed here under Client secret.
Deleting a user
You can delete a user in Intercom using the Suppression with Delete regulation of the RudderStack Data Regulation API.
userId
in the event. Additionally, you can specify a custom identifier (optional) in the event.A sample regulation request body for deleting a user in Intercom is shown below:
{ "regulationType": "suppress_with_delete", "destinationIds": [ "2FIKkByqn37FhzczP23eZmURciA" ], "users": [{ "userId": "1hKOmRA4GRlm", "<customKey>": "<customValue>" }]}
Track
The track
call lets you record the customer events, that is, the actions they perform, along with any properties associated with them.
A sample track
call is shown below:
rudderanalytics.track("Order Completed", { order_id: "140021222", checkout_id: "WAP3211", products: "sports_shoes" revenue: 77.95, currency: "USD",})
RudderStack converts and sends all the track
event properties as per the Intercom API.
Page
The page
call lets you record your website's page views with any additional relevant information about the viewed page.
The page
call is supported only for the JavaScript SDK when sending events via the device mode. It works by triggering Intercom's update
method, which looks for a list of new open conversations to be displayed to the current user.
A sample page
call looks like the following code snippet:
rudderanalytics.page("Best Seller")
Reset
The reset
method resets the previously identified user and any related information.
rudderClient.reset();
[[RSClient sharedInstance] reset];
FAQ
Does RudderStack support Intercom's push notification and deep linking features?
Unfortunately, none of the RudderStack SDKs support push notifications and deep linking features currently. Refer to the Intercom documentation for more information on configuring these features for your project.
What happens if both userId
or email
are missing in the identify
/ track
calls sent to Intercom?
For both identify
and track
calls, either userId
or email
is a mandatory field. In case both these fields are missing, RudderStack will drop the event.
It is highly recommended to enable the Send AnonymousId as Secondary UserId setting in your RudderStack dashboard to avoid any event loss in such a scenario.
Contact us
For more information on the topics covered on this page, email us or start a conversation in our Slack community.